Home
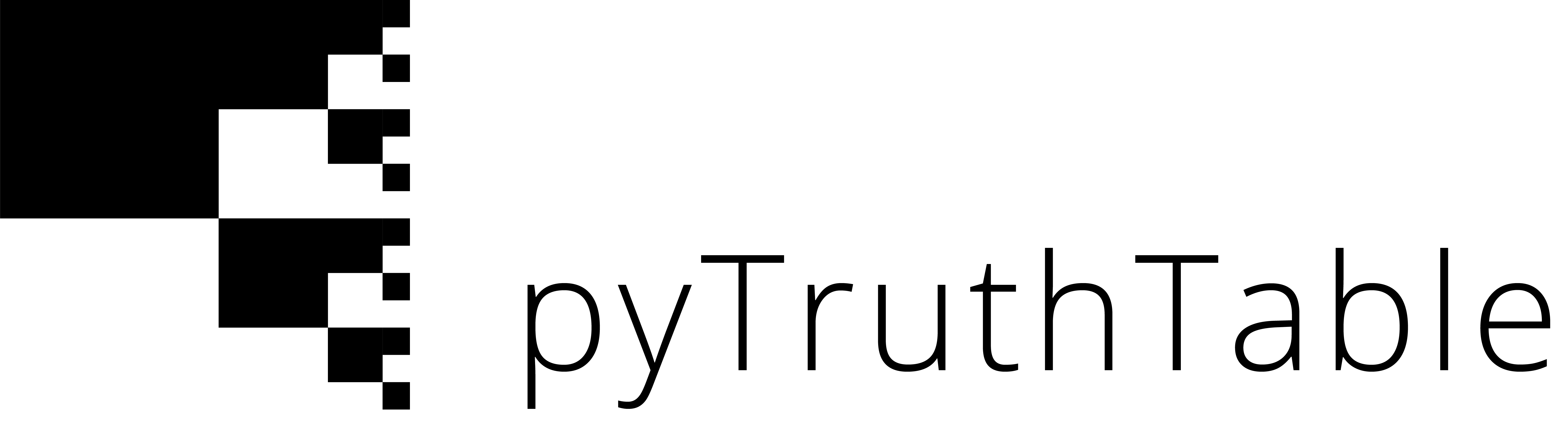
What is pyTruthTable?¶
pyTruthTable is a python library to create logical tables and make relations between its elements. It makes possible prepositional logic clauses analysis and binary operations to be used in classic logical problems.
Features:¶
- Uses Pandas Dataframe.
- Big set of logic operations.
- Simplified function calls.
- Automatic column naming.
- Customizible appearence.
Installation¶
Requirements: Python 3 and Pandas.
Install with Pypi:
pip3 install pyTruthTable
Take a look:¶
Example 1 - Binary operations¶
import pyTruthTable as ptt
# Initialize
t_table = ptt.PyTruthTable(["A", "B"])
# Create relations
t_table.append("not", "A")
t_table.append("and", "A", "B")
t_table.append("or", 2, "B") # Use the column index or name
t_table.append("xor", -2, -1)
t_table.append("nand", -1, 0, name = "C") # Rename column
t_table.append("equals", "C", "A")
A | B | ¬ A | A ^ B | ¬ A v B | (A ^ B) ⊕ (¬ A v B) | C | C ↔ A |
---|---|---|---|---|---|---|---|
True | True | False | True | True | False | True | True |
True | False | False | False | False | False | True | True |
False | True | True | False | True | True | True | False |
False | False | True | False | True | True | True | False |
Example 2 - Prepositional logic clauses¶
import pyTruthTable as ptt
# Initialize
tt = ptt.PyTruthTable(["Hot", "Wet", "Rains"])
# Append new column with specified operation
tt.append("and", "Hot", "Wet")
tt.append("implies", 3, "Rains")
Hot | Wet | Rains | Hot ^ Wet | (Hot ^ Wet) → (Rains) |
---|---|---|---|---|
True | True | True | True | True |
True | True | False | True | False |
True | False | True | False | True |
True | False | False | False | True |
False | True | True | False | True |
False | True | False | False | True |
False | False | True | False | True |
False | False | False | False | True |
See more examples here¶
Documentation & other links¶
- The amazing documentation is this Ṕage.
- Source code in the Github repository.
- Pypi pakage installer
License¶
Contributing is awesome!¶
See CONTRIBUTING
Contact¶
Developed with :heart: by Leonardo Mariga
leomariga@gmail.com
Did you like it? Remember to click on :star2: button.